jda-commands 4.0.0-beta.4 API
JDA-Commands
A declarative, annotation driven interaction framework for JDA.
Resources
This is the official documentation for jda-commands. If you are new to jda-commands (or JDA in general) you might also find the following resources helpful:
Having trouble or found a bug?
- Check out the Examples
- Join the Discord Server
- Or open an Issue
Getting JDA-Commands
Gradle
repositories {
mavenCentral()
}
dependencies {
implementation("io.github.kaktushose:jda-commands:VERSION")
}
Maven
<dependency>
<groupId>io.github.kaktushose</groupId>
<artifactId>jda-commands</artifactId>
<version>VERSION</version>
</dependency>
Example Usage
1. Entrypoint
This is the easiest way of starting JDA-Commands. Besides your JDA
(or ShardManager
) instance, we also need a
class of the classpath to scan for interactions.
public class Main {
public static void main(String[] args) {
JDA jda = yourJDABuilding();
JDACommands jdaCommands = JDACommands.start(jda, Main.class);
}
}
2. Defining Interactions
You define interactions as methods. They are made up from the method annotations and in some cases the method signature,
e.g. for command options. These methods must be contained in a class annotated with
Interaction
.
@Interaction
public class HelloWorld {
@SlashCommand("greet")
public void onCommand(CommandEvent event) {
event.reply("Hello World!");
}
}
Runtime Concept
One of the core concepts in jda-commands is the so-called Runtime
. It is mentioned frequently in the docs. A Runtime
delegates the jda events to their corresponding EventHandlers
and manages the used virtual threads.
A new Runtime
is created each time a SlashCommandInteractionEvent
,
GenericContextInteractionEvent
or CommandAutoCompleteInteractionEvent
is provided by jda
or if an interaction is marked as independent.
Runtimes are executed in parallel, but events are processed sequentially by each runtime.
Every EventHandler
called by this Runtime
is executed in its own virtual thread, isolated from the runtime one.
See ExpirationStrategy
for
details when a Runtime
will close.
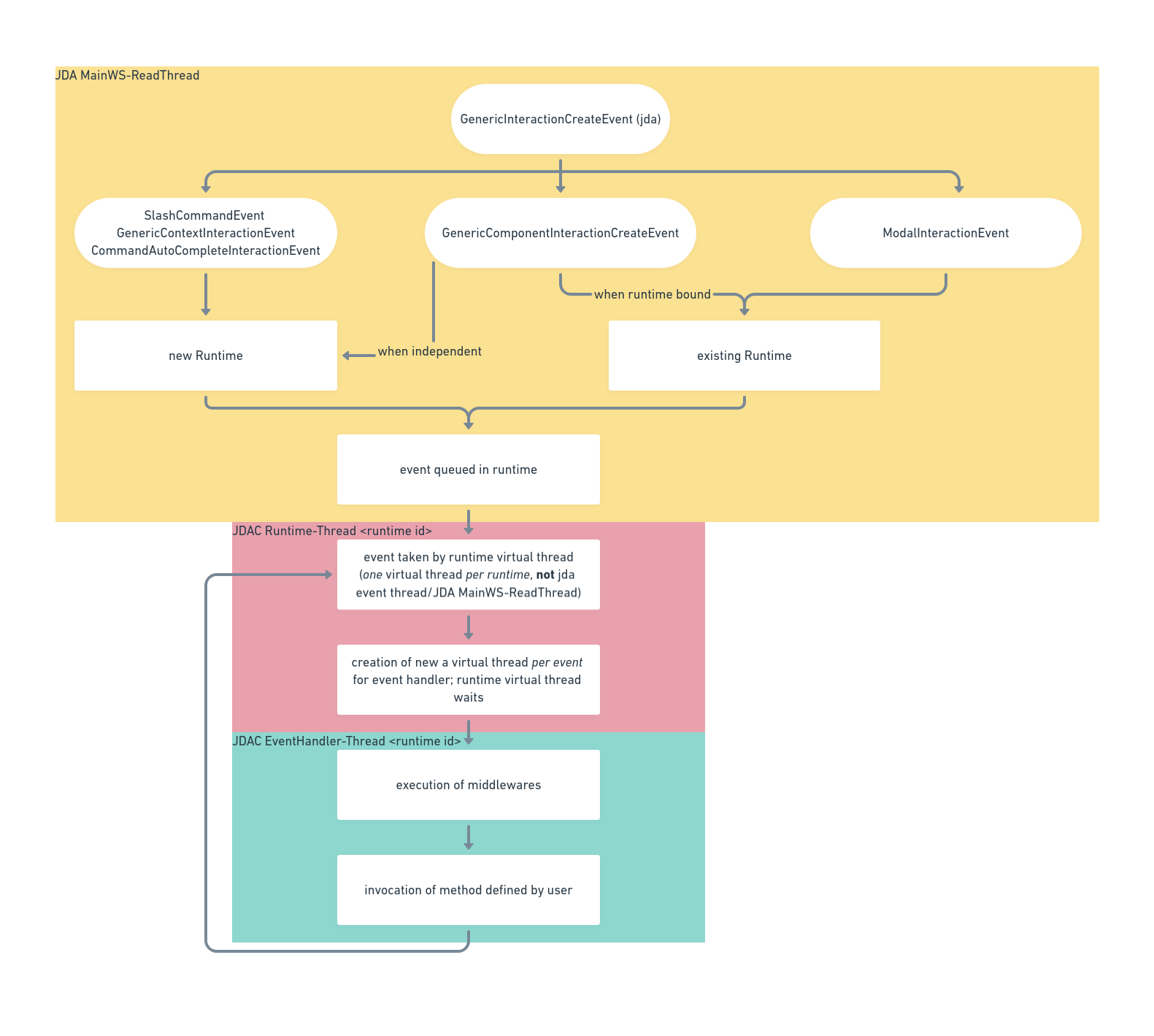
Project Structure
The JDA-Commands project is structured in two (JPMS/gradle) modules.
Name | JPMS Modules | Dependency |
---|---|---|
jda-commands | core + guice-extension | io.github.kaktushose:jda-commands:VERSION |
core | io.github.kaktushose.jda.commands.core | io.github.kaktushose.jda-commands:core:VERSION |
guice-extension | io.github.kaktushose.jda.commands.extension.guice | io.github.kaktushose.jda-commands:guice-extension:VERSION |
jda-commands
This is the module you get by using io.github.kaktushose:jda-commands:<version>
as a dependency.
It contains both the core
and guice-extension
module and is recommended for plug and play.
Attention: If you want to write an own extension, it should strictly depend on the core
module.
core
The core
module contains all general framework functionality, but lack a required dependency injection implementation.
guice-extension
The guice-extension
module provides Google's Guice as a dependency injection implementation, using JDA-Commands' own
extension system.
Guice Extension
This Extension
allows the use of Google Guice as a dependency injection framework.
It replaces the default InteractionClassInstantiator
implementation of the core module, which doesn't support
dependency injection, and configures Guice. See the Guice Wiki
for more information on how to use Guice.
Custom Injector
If you want to use a custom Guice Injector,
you can provide one by using GuiceExtensionData
.
...
Injector custom = Guice.createInjector();
JDACommands jdaCommands = JDACommands.builder(jda, Main.class)
.extensionData(new GuiceExtensionData(custom))
.start();
...
Automatically discovered implementations
This JDA-Commands Extension allows the automatic discovering of implementations of following interfaces:
- Middleware
- Validator
- TypeAdapter
- PermissionsProvider
- GuildScopeProvider
- ErrorMessageFactory
- Descriptor
To make these implementations discoverable please annotate the involved classes with @Implementation
.
If you're implementing a
TypeAdapter,
Middleware or
Validator
you have to provide additionally information in @Implementation
.
Please visit the docs of this class for further information.
Example
@Implementation(priority = Priority.NORMAL)
public class CustomMiddleware implements Middleware {
private static final Logger log = LoggerFactory.getLogger(FirstMiddleware.class);
@Override
public void accept(InvocationContext<?> context) {
log.info("run custom middleware");
}
}